Demystifying Marker Interfaces: A Simple Guide for Beginners
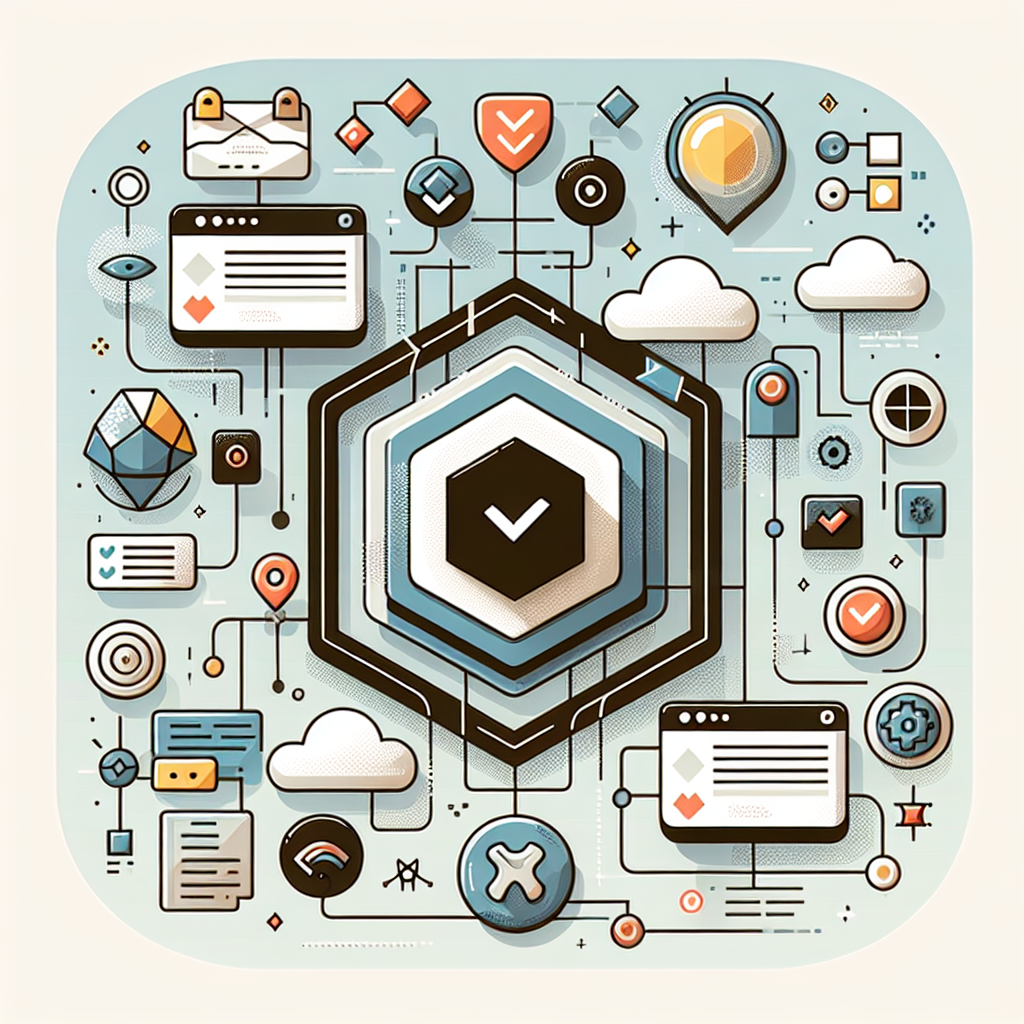
Understanding the Basics of Marker Interface: A Beginner’s Guide
If you’ve ever wondered what a marker interface is, don’t worry — you’re not alone! It’s a concept that’s often brushed over, but understanding it can add a neat trick to your coding toolkit. Let’s break it down into simple terms so you can grasp the essence without breaking a sweat.
What is a Marker Interface?
In the world of programming, a marker interface is an interface that doesn’t contain any methods or fields. Yeah, you read that right — it’s empty! But don’t let its emptiness fool you. Marker interfaces play a significant role, acting as a signal or a “marker” to the compiler that the objects of the implementing class have certain properties or should be treated a special way.
Think of it as a VIP badge at a concert. The badge itself doesn’t do anything physically, but it tells the event staff that you’re important and should be treated differently.
Key Points to Remember:
- Interface with No Methods: Just like plain water, a marker interface has no built-in flavor (methods or fields) but is essential for signaling.
- Implements Particular Behavior: While it doesn’t directly add behavior, it indicates that the class should be handled in a special manner.
- Example: In Java, interfaces like
Serializable
andCloneable
are typical markers.
Why Use a Marker Interface?
Using a marker interface can simplify certain checks and operations in your code. It provides a clean and standardized way of signaling that objects of a class are eligible for certain operations, like serialization.
For example, Java’s in-built serialization mechanism uses the Serializable
marker interface to check if an object can be serialized (converted into a byte stream).
Real-World Analogy:
Let’s say you’re at a theme park with an “Express Pass” (marker interface in disguise!). Although the pass doesn’t get you onto the roller coaster, it tells the park staff that you can jump the line and ride with fewer hassles!
How to Create a Marker Interface in Java
Creating a marker interface is as simple as defining an interface without methods. Here’s a quick example:
public interface MyMarkerInterface {
// No methods here, just marking!
}
public class MyClass implements MyMarkerInterface {
// Class implementation details
}
Adding a Node.js Perspective
While marker interfaces are a Java concept, similar ideas can be applied in JavaScript/Node.js using tagging patterns:
class MyMarkerClass {
// Just a placeholder for marking
}
class MyClass extends MyMarkerClass {
// Class logic
}
// Usage Check
let instance = new MyClass();
if (instance instanceof MyMarkerClass) {
console.log("Instance is marked!");
}
Closing Thoughts
To wrap it all up, a marker interface may seem like just an empty hoax, but it’s a powerful tool for signaling and organizing code. It lets you sprinkle some magic without adding complexity. Just remember, it’s like having a silent conversation with the JVM or your program’s logic, saying, “Hey, take special care of this!”
Now that you’re in on the secret, why not try implementing your own marker interfaces? Give it a whirl, and see how it can help in organizing your projects!